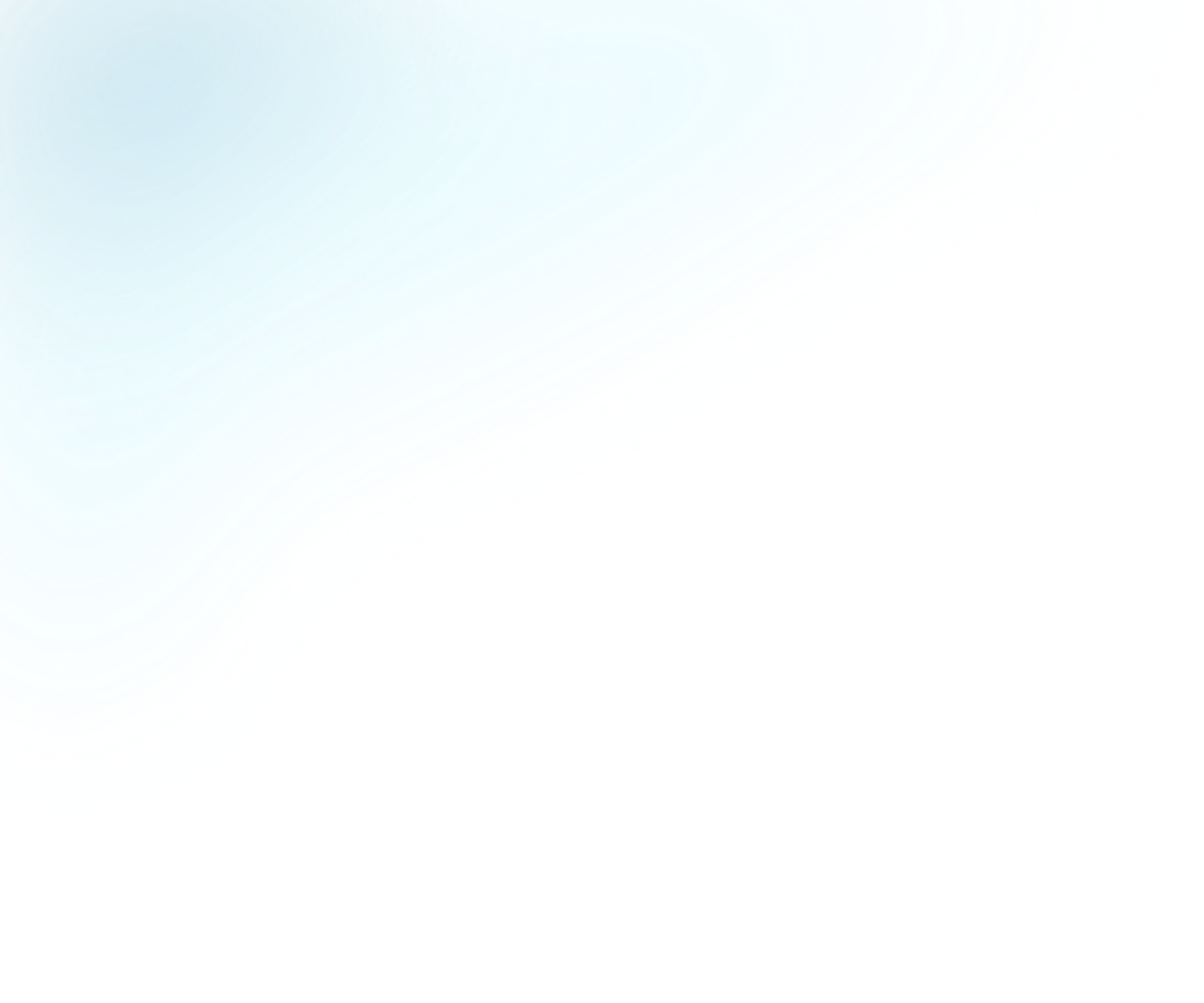
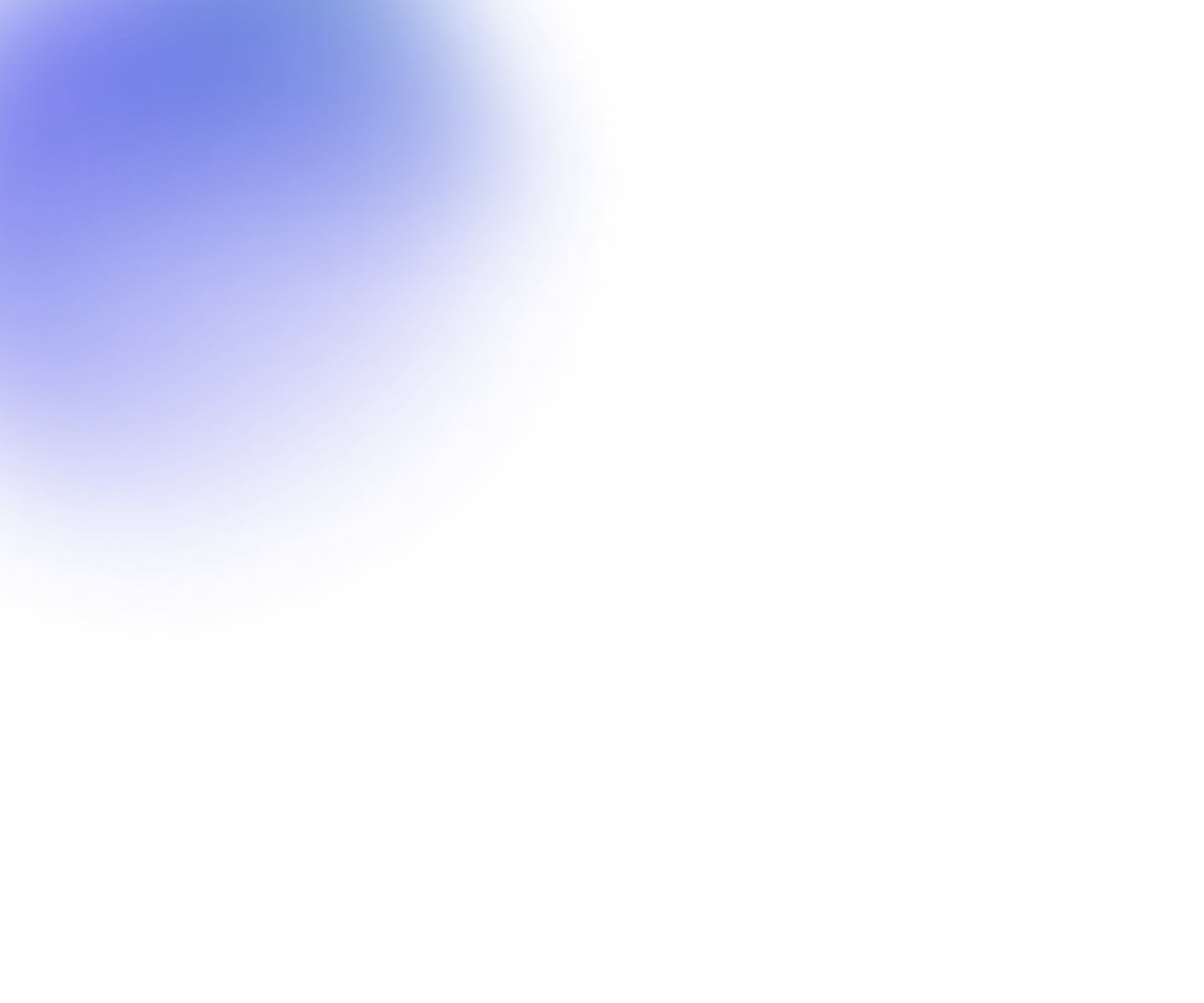
WebComponents
Introduction
WebComponents is a modern way of creating reusable components for browser based applications. Even though introduced already in 2011 the technology has started to gain traction just recently due to previously poor browser support. Now all major browsers have built-in support for the set of technologies needed to implement web components and thus they are ready for real life use cases.
How it works
WebComponents consist of three key technologies
- Custom elements
- Shadow DOM
- HTML Templates
To break this down Custom element defines the components behavior, shadow DOM allows you to style the component and with HTML templates you can define complex element structures in an easy way.
For instance you might want to have a component that shows some text below an image. This component could be called <image-with-caption>
. You could then use this component throughout your whole website by just using it as any other HTML tag.
body {
background-color: #fff;
padding: 2em;
}
image-with-caption {
max-width: 20em;
display: block;
}
image-with-caption img {
display: block;
width: 100%;
}
image-with-caption span {
display: block;
padding-left: 2em;
font-style: italic;
}
class ImageWithCaption extends HTMLElement {
constructor() {
super()
this.renderImage()
this.renderCaption()
}
renderImage() {
const img = document.createElement("img")
img.src = this.getAttribute("src")
this.appendChild(img)
}
renderCaption() {
const caption = document.createElement("span")
caption.innerHTML = this.getAttribute("caption")
this.appendChild(caption)
}
}
customElements.define("image-with-caption", ImageWithCaption)
WebComponents at Flowplayer
At Flowplayer we have always wanted to allow player customization as far as possible. We have provided powerful APIs for scripting and allowed a versatile set of CSS classes for appearance modifications.
In Flowplayer Native v3 we wanted to take customization to the next level. We headed off to rewrite the whole UI layer with WebComponents. At the same time we created a registry for the player that contains all the components the player uses to build the UI. Everything in the player UI is a component - from icons to more complex structures like menus and sliders.
Every component can be replaced with your own implementation. Want your icons to look different - replace the icon component. Want your volume bar vertical instead of horizontal - replace the volume slider component! Even the topmost UI wrapper is a component. This means that if you want to roll out a completely own UI you can just replace the top level component with your own and we won’t render our default UI at all! And as the cherry on top of the cake - in your own implementation you can reuse all our components if you want to.
This small example below shows how easy it is to replace the header component and style it and access the player API handle inside the component.
class CustomHeader extends HTMLElement {
constructor(player) {
super()
this.player = player
this.render()
this.player.on(flowplayer.events.DURATION_CHANGE, () => this.render())
}
render() {
const title = this.player.opt("title")
const description = this.player.opt("description")
this.innerHTML = `This video has a title "${title}" and description "${description}" and it is ${this.duration() || '?'} seconds long. This header is a custom WebComponent.`
}
duration() {
return (this.player.duration || 0).toFixed(2)
}
}
customElements.define("custom-header", CustomHeader)
flowplayer.customElements.set("flowplayer-header", "custom-header")
const player = flowplayer("#player",
{
title: "My video",
desc: "My description",
src: "https://cdn.flowplayer.com/d9cd469f-14fc-4b7b-a7f6-ccbfa755dcb8/hls/383f752a-cbd1-4691-a73f-a4e583391b3d/playlist.m3u8"
})
.flowplayer custom-header {
background-color: rgba(0,0,0,0.7);
padding: 2em;
}
To learn more about WebComponents in general you should head to MDN documentation
To learn more about WebComponents at Flowplayer head to our WebComponents documentation